
随着计算机和互联网的出现,大量信息以数字方式获取。不同的公司想出了解决方案来提高这个过程的效率。一种这样的解决方案是可填写的 PDF 表格。 PDF 表单是一种流行的选择,可以轻松地以数字方式捕获信息。 PDF 表格可用于捕获调查数据或作为录取表格。鉴于此,本文将教您如何使用 C++ 创建、填写和编辑可填写的 PDF 表单。
- 用于创建、填写和编辑可填写 PDF 表单的 C++ API
- 使用 C++ 创建可填写的 PDF 表单
- 使用 C++ 在 PDF 文件中填写现有表格
- 使用 C++ 修改 PDF 表单中表单字段的值
- 使用 C++ 从现有 PDF 表单中删除表单域
用于创建、填写和编辑可填写 PDF 表单的 C++ API
Aspose.PDF for C++ 是一个 C++ 库,允许您创建、阅读和更新 PDF 文档。此外,该 API 支持创建、填写和编辑可填写的 PDF 表单。您可以通过 NuGet 安装 API,也可以直接从 下载 部分下载。
PM> Install-Package Aspose.PDF.Cpp
使用 C++ 创建可填写的 PDF 表单
在此示例中,我们将从头开始创建一个带有两个文本框和一个单选按钮的表单。但是,一个文本框是多行的,另一个是单行的。以下是在 PDF 文件中创建表单的步骤。
- 创建 Document 类的实例。
- 在文档中添加一个空白页。
- 创建 TextBoxField 类的实例。
- 设置 TextBoxField 的属性,如 FontSize、Color 等。
- 创建第二个 TextBoxField 的实例并设置其属性。
- 使用 Document->getForm()->Add(System::SharedPtr) 将两个文本框添加到表单中字段,int32t pageNumber) 方法。
- 创建一个表。
- 创建 RadioButtonField 类的实例。
- 使用 Document->getForm()->Add(System::SharedPtr) 将 RadioButton 添加到表单字段,int32t pageNumber) 方法。
- 创建 RadioButtonOptionField 类的两个实例来表示单选按钮的选项。
- 设置 OptionName、Width 和 Height 并使用 RadioButtonField->Add(System::SharedPtr) 将选项添加到单选按钮const & newItem) 方法。
- 使用 Cell->getParagraphs()->Add(System::SharedPtr) 将单选按钮的选项添加到表格的单元格段) 方法。
- 使用 Document->Save(System::String outputFileName) 方法保存输出文件。
以下示例代码展示了如何使用 C++ 在 PDF 文件中创建表单。
// 创建 Document 类的实例
auto pdfDocument = MakeObject<Document>();
// 向文档添加空白页
System::SharedPtr<Page> page = pdfDocument->get_Pages()->Add();
System::SharedPtr<Aspose::Pdf::Rectangle> rectangle1 = MakeObject<Aspose::Pdf::Rectangle>(275, 740, 440, 770);
// 创建一个文本框字段
System::SharedPtr<TextBoxField> nameBox = MakeObject<TextBoxField>(pdfDocument, rectangle1);
nameBox->set_PartialName(u"nameBox1");
nameBox->get_DefaultAppearance()->set_FontSize(10);
nameBox->set_Multiline(true);
System::SharedPtr<Border> nameBorder = MakeObject<Border>(nameBox);
nameBorder->set_Width(1);
nameBox->set_Border(nameBorder);
nameBox->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());
nameBox->set_Color(Aspose::Pdf::Color::FromRgb(System::Drawing::Color::get_Red()));
System::SharedPtr<Aspose::Pdf::Rectangle> rectangle2 = MakeObject<Aspose::Pdf::Rectangle>(275, 718, 440, 738);
// 创建一个文本框字段
System::SharedPtr<TextBoxField> mrnBox = MakeObject<TextBoxField>(pdfDocument, rectangle2);
mrnBox->set_PartialName(u"Box1");
mrnBox->get_DefaultAppearance()->set_FontSize(10);
System::SharedPtr<Border> mrnBorder = MakeObject<Border>(mrnBox);
mrnBox->set_Width(165);
mrnBox->set_Border(mrnBorder);
mrnBox->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());
mrnBox->set_Color(Aspose::Pdf::Color::FromRgb(System::Drawing::Color::get_Red()));
// 将 TextBoxField 添加到表单
pdfDocument->get_Form()->Add(nameBox, 1);
pdfDocument->get_Form()->Add(mrnBox, 1);
// 创建表
System::SharedPtr<Table> table = MakeObject<Table>();
table->set_Left(200);
table->set_Top(300);
table->set_ColumnWidths(u"120");
// 将表格添加到页面
page->get_Paragraphs()->Add(table);
// 创建行和列
System::SharedPtr<Row> r1 = table->get_Rows()->Add();
System::SharedPtr<Row> r2 = table->get_Rows()->Add();
System::SharedPtr<Cell> c1 = r1->get_Cells()->Add();
System::SharedPtr<Cell> c2 = r2->get_Cells()->Add();
// 创建一个 RadioButtonField
System::SharedPtr<RadioButtonField> rf = MakeObject<RadioButtonField>(page);
rf->set_PartialName(u"radio");
// 将 RadioButtonField 添加到表单
pdfDocument->get_Form()->Add(rf, 1);
// 创建单选按钮选项
System::SharedPtr<RadioButtonOptionField> opt1 = MakeObject<RadioButtonOptionField>();
System::SharedPtr<RadioButtonOptionField> opt2 = MakeObject<RadioButtonOptionField>();
opt1->set_OptionName(u"Yes");
opt2->set_OptionName(u"No");
opt1->set_Width(15);
opt1->set_Height(15);
opt2->set_Width(15);
opt2->set_Height(15);
// 将选项添加到 RadioButtonField
rf->Add(opt1);
rf->Add(opt2);
System::SharedPtr<Border> opt1Border = MakeObject<Border>(opt1);
opt1->set_Border(opt1Border);
opt1->get_Border()->set_Width(1);
opt1->get_Border()->set_Style(BorderStyle::Solid);
opt1->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());
opt1->get_DefaultAppearance()->set_TextColor(System::Drawing::Color::get_Red());
System::SharedPtr<TextFragment> opt1Fragment = MakeObject<TextFragment>(u"Yes");
opt1->set_Caption(opt1Fragment);
System::SharedPtr<Border> opt2Border = MakeObject<Border>(opt2);
opt2->set_Border(opt2Border);
opt2->get_Border()->set_Width(1);
opt2->get_Border()->set_Style(BorderStyle::Solid);
opt2->get_Characteristics()->set_Border(System::Drawing::Color::get_Black());
opt2->get_DefaultAppearance()->set_TextColor(System::Drawing::Color::get_Red());
System::SharedPtr<TextFragment> opt2Fragment = MakeObject<TextFragment>(u"No");
opt2->set_Caption(opt2Fragment);
// 将选项添加到表格的单元格
c1->get_Paragraphs()->Add(opt1);
c2->get_Paragraphs()->Add(opt2);
// 保存输出文件
pdfDocument->Save(u"OutputDirectory\\Fillable_PDF_Form_Out.pdf");
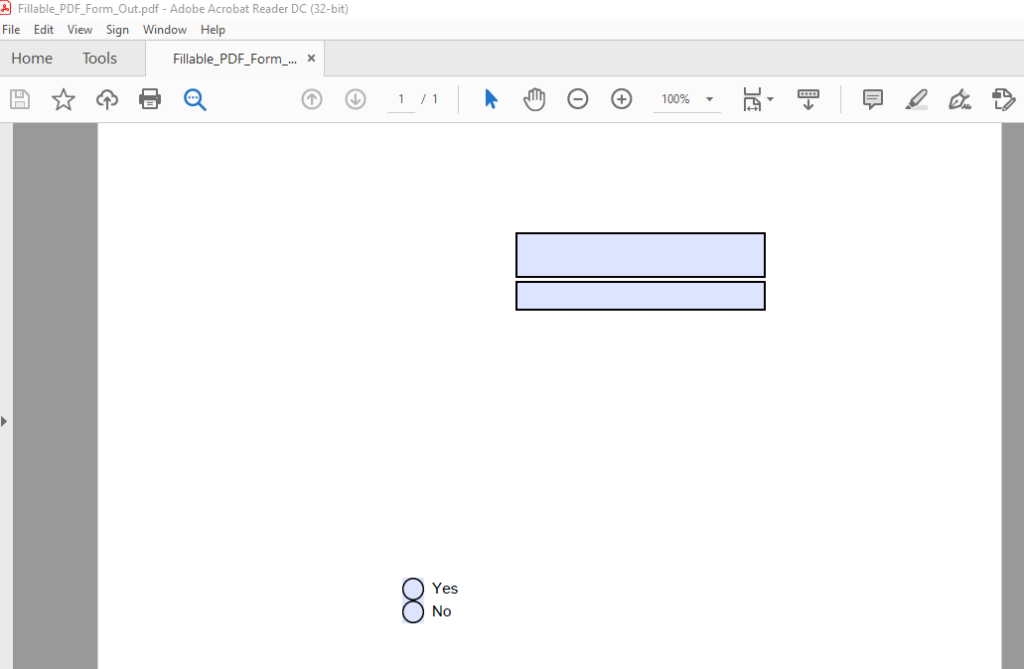
示例代码生成的 PDF 文件的图像
使用 C++ 在 PDF 文件中填写现有表格
在此示例中,我们将使用上一个示例中生成的文件。我们将使用 Document 类加载文件并填写其字段。以下是填写现有 PDF 表单字段的步骤。
- 使用 Document 类加载 PDF 文件。
- 使用 Document->getForm()->idxget(System::String name) 方法检索 TextBoxFields。
- 使用 TextBoxField->setValue(System::String value) 方法设置两个 TextBoxField 的值。
- 使用 Document->getForm()->idxget(System::String name) 方法检索 RadioButtonField。
- 使用 RadioButtonField->setSelected(int32t value) 方法设置 RadioButtonField 的值。
- 使用 Document->Save(System::String outputFileName) 方法保存输出文件。
以下示例代码展示了如何使用 C++ 在 PDF 文件中填写现有表单。
// 加载 PDF 文件
auto pdfDocument = MakeObject<Document>(u"SourceDirectory\\Fillable_PDF_Form.pdf");
// 检索文本框字段
System::SharedPtr<TextBoxField> textBoxField1 = System::DynamicCast<TextBoxField>(pdfDocument->get_Form()->idx_get(u"nameBox1"));
System::SharedPtr<TextBoxField> textBoxField2 = System::DynamicCast<TextBoxField>(pdfDocument->get_Form()->idx_get(u"Box1"));
// 设置文本框字段的值
textBoxField1->set_Value(u"A quick brown fox jumped over the lazy dog.");
textBoxField2->set_Value(u"A quick brown fox jumped over the lazy dog.");
// 检索单选按钮字段
System::SharedPtr<RadioButtonField> radioField = System::DynamicCast<RadioButtonField>(pdfDocument->get_Form()->idx_get(u"radio"));
// 设置单选按钮字段的值
radioField->set_Selected(1);
// 保存输出文件
pdfDocument->Save(u"OutputDirectory\\Fill_PDF_Form_Field_Out.pdf");

示例代码生成的 PDF 文件的图像
使用 C++ 修改 PDF 表单中表单字段的值
使用 Aspose.PDF for C++,我们还可以修改之前填写的字段的值。在本例中,我们将使用上例中生成的文件,并修改第一个 TextBoxField 的值。为此,请按照以下步骤操作。
- 使用 Document 类加载 PDF 文件。
- 使用 Document->getForm()->idxget(System::String name) 方法检索 TextBoxField。
- 使用 TextBoxField->setValue(System::String value) 方法更新 TextBoxField 的值。
- 使用 Document->Save(System::String outputFileName) 方法保存输出文件。
以下示例代码展示了如何使用 C++ 修改 PDF 表单中的字段值。
// 加载 PDF 文件
auto pdfDocument = MakeObject<Document>(u"SourceDirectory\\Fill_PDF_Form_Field.pdf");
// 检索 TextBoxField
System::SharedPtr<TextBoxField> textBoxField = System::DynamicCast<TextBoxField>(pdfDocument->get_Form()->idx_get(u"nameBox1"));
// 更新 TextBoxField 的值
textBoxField->set_Value(u"Changed Value");
// 将 TextBoxField 标记为只读
textBoxField->set_ReadOnly(true);
// 保存输出文件
pdfDocument->Save(u"OutputDirectory\\Modify_Form_Field_out.pdf");

示例代码生成的 PDF 文件的图像
使用 C++ 从现有 PDF 表单中删除表单域
该 API 还允许您从现有 PDF 表单中删除表单域。以下是从 PDF 表单中删除表单域的步骤。
- 使用 Document 类加载 PDF 文件。
- 使用 Document->getForm()->Delete(System::String fieldName) 方法删除字段。
- 使用 Document->Save(System::String outputFileName) 方法保存输出文件。
以下示例代码展示了如何使用 C++ 从现有 PDF 表单中删除表单域。
// 加载 PDF 文件
auto pdfDocument = MakeObject<Document>(u"SourceDirectory\\Fill_PDF_Form_Field.pdf");
// 删除字段
pdfDocument->get_Form()->Delete(u"nameBox1");
// 保存输出文件
pdfDocument->Save(u"OutputDirectory\\Delete_Form_Field_out.pdf");
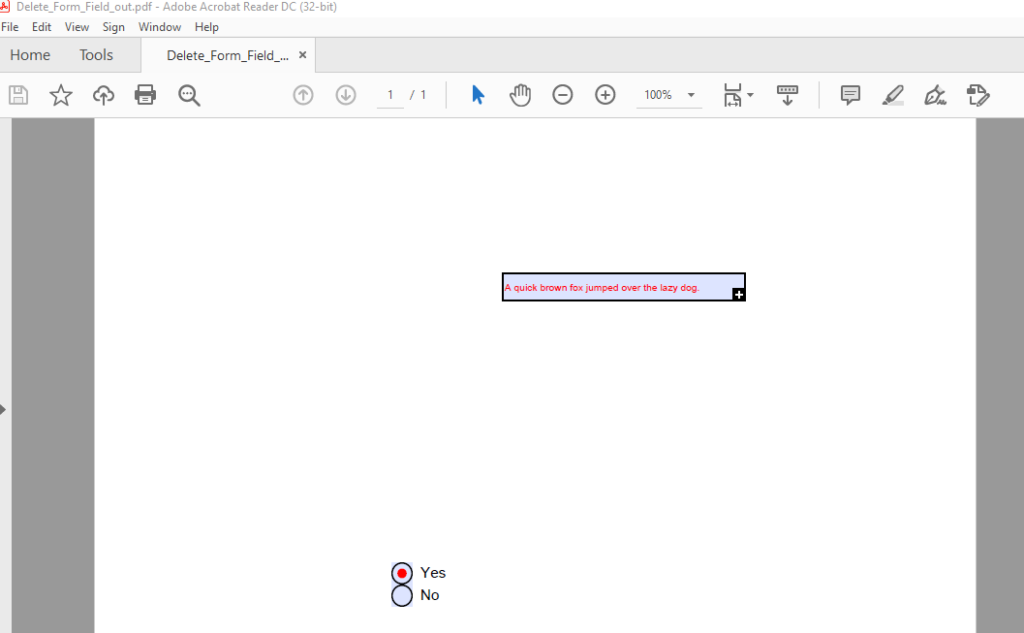
示例代码生成的 PDF 文件的图像
获得免费许可证
您可以通过申请 免费的临时许可证 来试用该 API,而不受评估限制。
结论
在本文中,您学习了如何使用 C++ 在 PDF 文件中创建表单。此外,您还学习了如何填写和修改 PDF 表单中的现有字段。您还了解了如何使用 Aspose.PDF for C++ API 从 PDF 表单中删除表单域。 API 提供了一系列用于处理 PDF 文件的附加功能,您可以通过 官方文档 详细了解这些功能。如果您对 API 的任何方面有任何疑问,请随时通过我们的 免费支持论坛 与我们联系。