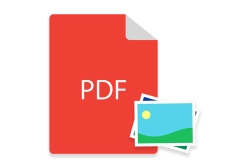
一张图片胜过千言万语。因此,图像和图形在 PDF 以及其他文档中发挥着重要作用。由于 PDF 已成为最流行和广泛使用的文件格式之一,本文主要介绍如何以编程方式处理 PDF 文件中的图像。更准确地说,您将学习如何在 C# .NET 中从 PDF 文件中添加、提取、删除和替换图像。
- C# .NET API 用于处理 PDF 中的图像
- 使用 C# 在 PDF 中添加图像
- 使用 C# 从 PDF 中提取图像
- 使用 C# 从 PDF 中删除图像
- 使用 C# 替换 PDF 中的图像
- 获得免费许可证
用于在 PDF 中添加、删除和替换图像的 C# API - 免费下载
Aspose.PDF for .NET 是一个 C# 类库,可让您从 .NET 应用程序中创建和操作 PDF 文档。使用 API,您可以非常轻松地执行基本和高级 PDF 自动化功能。此外,您可以处理现有 PDF 文件中的图像。 API 可以作为 DLL 下载或通过 NuGet 安装。
PM> Install-Package Aspose.Pdf
在 C# .NET 中的 PDF 文件中添加图像
以下是使用 Aspose.PDF for .NET 将图像添加到 PDF 文件的步骤。
- 使用 Document 类创建新的或加载现有的 PDF 文件。
- 在 Page 对象中获取所需页面的引用。
- 将图像添加到页面的 Resources 集合中。
- 使用以下运算符将图像放置在页面上:
- 使用 Document.Save(String) 方法保存更新的 PDF 文件。
以下代码示例展示了如何使用 C# 将图像添加到 PDF 文件。
// 如需完整的示例和数据文件,请访问 https://github.com/aspose-pdf/Aspose.PDF-for-.NET
// 打开文档
Document pdfDocument = new Document("AddImage.pdf");
// 设置坐标
int lowerLeftX = 100;
int lowerLeftY = 100;
int upperRightX = 200;
int upperRightY = 200;
// 获取需要添加图片的页面
Page page = pdfDocument.Pages[1];
// 将图像加载到流中
FileStream imageStream = new FileStream("aspose-logo.jpg", FileMode.Open);
// 将图像添加到页面资源的图像集合
page.Resources.Images.Add(imageStream);
// 使用 GSave 运算符:此运算符保存当前图形状态
page.Contents.Add(new Aspose.Pdf.Operators.GSave());
// 创建矩形和矩阵对象
Aspose.Pdf.Rectangle rectangle = new Aspose.Pdf.Rectangle(lowerLeftX, lowerLeftY, upperRightX, upperRightY);
Matrix matrix = new Matrix(new double[] { rectangle.URX - rectangle.LLX, 0, 0, rectangle.URY - rectangle.LLY, rectangle.LLX, rectangle.LLY });
// 使用 ConcatenateMatrix(连接矩阵)运算符:定义图像必须如何放置
page.Contents.Add(new Aspose.Pdf.Operators.ConcatenateMatrix(matrix));
XImage ximage = page.Resources.Images[page.Resources.Images.Count];
// 使用 Do 运算符:此运算符绘制图像
page.Contents.Add(new Aspose.Pdf.Operators.Do(ximage.Name));
// 使用 GRestore 运算符:此运算符恢复图形状态
page.Contents.Add(new Aspose.Pdf.Operators.GRestore());
// 保存更新的文档
pdfDocument.Save("AddImage_out.pdf");
在 C# 中从 PDF 中提取图像
如果您想从 PDF 文件中提取所有图像,您可以按照以下步骤操作。
- 使用 Document 类加载现有的 PDF 文件。
- 使用索引从特定页面的 Resources 集合中获取 XImage 对象中所需的图像。
- 使用 XImage.Save(FileStream, ImageFormat) 方法将提取的图像保存为所需的格式。
以下代码示例展示了如何使用 C# 从 PDF 中提取图像。
// 如需完整的示例和数据文件,请访问 https://github.com/aspose-pdf/Aspose.PDF-for-.NET
// 打开文档
Document pdfDocument = new Document("ExtractImages.pdf");
// 提取特定图像
XImage xImage = pdfDocument.Pages[1].Resources.Images[1];
FileStream outputImage = new FileStream("output.jpg", FileMode.Create);
// 保存输出图像
xImage.Save(outputImage, ImageFormat.Jpeg);
outputImage.Close();
在 C# 中从 PDF 中删除图像
一旦您可以访问 PDF 页面的资源,您就可以从中删除图像。以下是使用 C# 从 PDF 文件中删除图像的步骤。
- 使用 Document 类加载 PDF 文件。
- 使用以下方法之一删除图像。
- 使用 Document.Save(String) 方法保存更新的 PDF 文件。
以下代码示例展示了如何使用 C# 从 PDF 中删除图像。
// 如需完整的示例和数据文件,请访问 https://github.com/aspose-pdf/Aspose.PDF-for-.NET
// 打开文档
Document pdfDocument = new Document("DeleteImages.pdf");
// 删除特定图像
pdfDocument.Pages[1].Resources.Images.Delete(1);
// 保存更新的 PDF 文件
pdfDocument.Save("output.pdf");
在 C# 中替换 PDF 中的图像
Aspose.PDF for .NET 还允许您替换 PDF 中的特定图像。为此,您可以替换页面图像集中的图像。以下是使用 C# 替换 PDF 中的图像的步骤。
- 使用 Document 类加载 PDF 文件。
- 使用 [Document.Pages1.Resources.Images.Replace(Int32, Stream, Int32, Boolean)]24 方法替换所需的图像。
- 使用 Document.Save(String) 方法保存更新的 PDF 文件。
以下代码示例展示了如何使用 C# 替换 PDF 中的图像。
// 如需完整的示例和数据文件,请访问 https://github.com/aspose-pdf/Aspose.PDF-for-.NET
// 打开文档
Document pdfDocument = new Document("input.pdf");
// 替换特定图像
pdfDocument.Pages[1].Resources.Images.Replace(1, new FileStream("lovely.jpg", FileMode.Open));
// 保存更新的 PDF 文件
pdfDocument.Save("output.pdf");
C# .NET PDF API - 获得免费许可证
您可以 获得免费的临时许可证 以便在没有评估限制的情况下试用 API。
结论
图像和图形对象是 PDF 文档的重要元素。因此,在本文中,我们介绍了如何使用 C# .NET API 处理 PDF 中的图像。分步教程和代码示例展示了如何在 C# 中添加、提取、删除和替换 PDF 文件中的图像。您可以使用 文档 探索有关 C# PDF API 的更多信息。