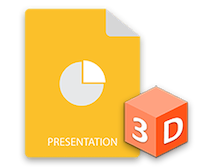
PowerPoint 中的 3D 效果用于使演示文稿更具吸引力并吸引用户的注意力因此,您可能会遇到以编程方式将 3D 对象添加到演示文稿的需求。在本文中,您将学习如何在 PowerPoint PPT 或 Java 中的 PPTX 中创建 3D 效果。我们将向您展示如何创建 3D 文本和形状并将 3D 效果应用于图像。
- 在 PowerPoint 中创建 3D 效果的 Java API
- 用 Java 在 PowerPoint 中创建 3D 文本
- 在 Java 中的 PowerPoint 中创建 3D 形状
- 为 3D 形状设置渐变
- 将 3D 效果应用于 PowerPoint 中的图像
Java API 在 PowerPoint PPT 中应用 3D 效果
Aspose.Slides for Java 是一个强大的 API,封装了广泛的演示操作功能。使用 API,您可以创建交互式演示文稿并无缝操作现有的 PPT/PPTX 文件。为了在 PowerPoint 演示文稿中创建 3D 效果,我们将使用此 API。
您可以 下载 API 的 JAR 或使用以下 Maven 配置安装它。
存储库:
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://repository.aspose.com/repo/</url>
</repository>
依赖:
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>22.1</version>
<classifier>jdk16</classifier>
</dependency>
用 Java 在 PowerPoint 中创建 3D 文本
以下是使用 Java 在 PowerPoint PPT 中创建 3D 文本片段的步骤。
- 首先,创建一个新的 PPT 或使用 Presentation 类加载现有的。
- 然后,使用 addAutoShape() 方法添加一个新的矩形形状。
- 设置形状的属性,例如填充类型、文本等。
- 将形状内的文本引用到 Portion 对象中。
- 将格式应用于文本部分。
- 获取 TextFrame 内部形状的参考。
- 使用 TextFrame.getTextFrameFormat().getThreeDFormat() 方法返回的 IThreeDFormat 应用 3D 效果。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
下面的代码示例展示了如何在 Java 的 PowerPoint PPT 中创建 3D 文本。
// 创建演示文稿
Presentation pres = new Presentation();
try {
// 添加矩形形状
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// 设置文本
shape.getFillFormat().setFillType(FillType.NoFill);
shape.getLineFormat().getFillFormat().setFillType(FillType.NoFill);
shape.getTextFrame().setText("3D Text");
// 添加文本部分并设置其属性
Portion portion = (Portion)shape.getTextFrame().getParagraphs().get_Item(0).getPortions().get_Item(0);
portion.getPortionFormat().getFillFormat().setFillType(FillType.Pattern);
portion.getPortionFormat().getFillFormat().getPatternFormat().getForeColor().setColor(new Color(255, 140, 0));
portion.getPortionFormat().getFillFormat().getPatternFormat().getBackColor().setColor(Color.WHITE);
portion.getPortionFormat().getFillFormat().getPatternFormat().setPatternStyle(PatternStyle.LargeGrid);
// 设置形状文本的字体大小
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(128);
// 获取文本框
ITextFrame textFrame = shape.getTextFrame();
// 设置“Arch Up”艺术字变换效果
textFrame.getTextFrameFormat().setTransform(TextShapeType.ArchUp);
// 应用 3D 效果
textFrame.getTextFrameFormat().getThreeDFormat().setExtrusionHeight(3.5f);
textFrame.getTextFrameFormat().getThreeDFormat().setDepth(3);
textFrame.getTextFrameFormat().getThreeDFormat().setMaterial(MaterialPresetType.Plastic);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Balanced);
textFrame.getTextFrameFormat().getThreeDFormat().getLightRig().setRotation(0, 0, 40);
textFrame.getTextFrameFormat().getThreeDFormat().getCamera().setCameraType(CameraPresetType.PerspectiveContrastingRightFacing);
// 保存演示文稿
pres.save("3D-Text.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
以下屏幕截图显示了上述代码示例的输出。
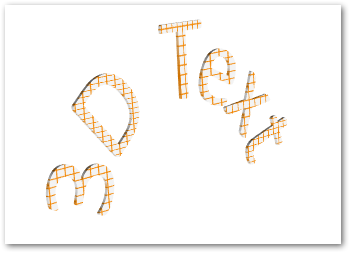
在 Java 中的 PowerPoint 中创建 3D 形状
与文本类似,您可以将 3D 效果应用于 PowerPoint 演示文稿中的形状。以下是使用 Java 在 PowerPoint PPT 中创建 3D 形状的步骤。
- 首先,使用Presentation类创建一个新的PPT。
- 使用 addAutoShape() 方法添加一个新的矩形形状。
- 使用 IAutoShape.getTextFrame.setText() 方法设置形状的文本。
- 使用 IAutoShape.getThreeDFormat() 方法返回的 IThreeDFormat 将 3D 效果应用于形状。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
以下代码示例演示如何使用 Java 将 3D 效果应用于 PowerPoint 中的形状。
// 创建演示文稿
Presentation pres = new Presentation();
try {
// 添加矩形形状
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// 为形状设置文本
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
// 应用 3D 效果
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(20, 30, 40);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setMaterial(MaterialPresetType.Flat);
shape.getThreeDFormat().setExtrusionHeight(100);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.BLUE);
// 保存演示文稿
pres.save("3D-Shape.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
下面是我们执行这段代码后得到的 3D 形状。
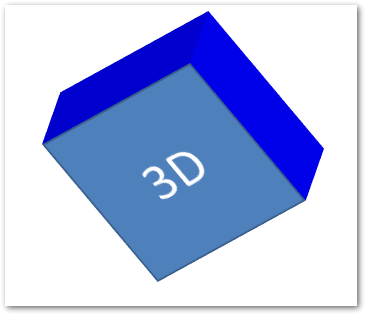
为 3D 形状创建渐变
您还可以按照以下步骤将渐变效果应用于形状。
- 首先,使用 Presentation 类创建一个新的 PPT。
- 使用 addAutoShape() 方法添加一个新的矩形形状。
- 使用 IAutoShape.getTextFrame().setText() 属性设置形状的文本。
- 将形状的填充类型设置为 FillType.Gradient 并设置渐变颜色。
- 使用 IAutoShape.getThreeDFormat() 方法返回的 IThreeDFormat 将 3D 效果应用于形状。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
以下代码示例演示如何将渐变效果应用于 PowerPoint PPT 中的形状。
// 创建演示文稿
Presentation pres = new Presentation();
try {
// 添加矩形形状
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// 为形状设置文本
shape.getTextFrame().setText("3D");
shape.getTextFrame().getParagraphs().get_Item(0).getParagraphFormat().getDefaultPortionFormat().setFontHeight(64);
shape.getFillFormat().setFillType(FillType.Gradient);
shape.getFillFormat().getGradientFormat().getGradientStops().add(0, Color.BLUE);
shape.getFillFormat().getGradientFormat().getGradientStops().add(100, Color.MAGENTA);
// 应用 3D 效果
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(new Color(255, 140, 0));
// 保存演示文稿
pres.save("3D-Shape-Gradient.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
以下是应用渐变效果后的 3D 形状。
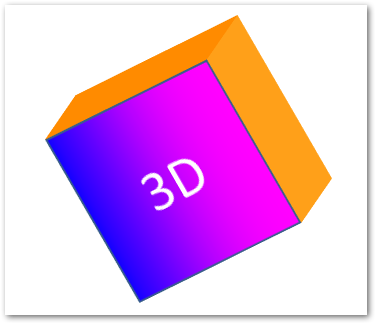
在 Java 中的 PowerPoint 中将 3D 效果应用于图像
Aspose.Slides for Java 还允许您将 3D 效果应用于图像。以下是在 Java 中执行此操作的步骤。
- 使用 Presentation 类创建一个新的 PPT。
- 使用 addAutoShape() 方法添加一个新的矩形形状。
- 将形状的填充类型设置为 FillType.Picture 并添加图像。
- 使用 IAutoShape.getThreeDFormat() 方法返回的 IThreeDFormat 将 3D 效果应用于形状。
- 使用 Presentation.save(String, SaveFormat) 方法保存演示文稿。
以下是使用 Java 对 PPT 中的图像应用 3D 效果的步骤。
// 创建演示文稿
Presentation pres = new Presentation();
try {
// 添加矩形形状
IAutoShape shape = pres.getSlides().get_Item(0).getShapes().addAutoShape(ShapeType.Rectangle, 200, 150, 200, 200);
// 为形状设置图像
shape.getFillFormat().setFillType(FillType.Picture);
IPPImage picture = null;
try {
picture = pres.getImages().addImage(Files.readAllBytes(Paths.get("tiger.bmp")));
} catch (IOException e) { }
shape.getFillFormat().getPictureFillFormat().getPicture().setImage(picture);
shape.getFillFormat().getPictureFillFormat().setPictureFillMode(PictureFillMode.Stretch);
// 应用 3D 效果
shape.getThreeDFormat().getCamera().setCameraType(CameraPresetType.OrthographicFront);
shape.getThreeDFormat().getCamera().setRotation(10, 20, 30);
shape.getThreeDFormat().getLightRig().setLightType(LightRigPresetType.Flat);
shape.getThreeDFormat().getLightRig().setDirection(LightingDirection.Top);
shape.getThreeDFormat().setExtrusionHeight(150);
shape.getThreeDFormat().getExtrusionColor().setColor(Color.GRAY);
// 保存演示文稿
pres.save("3D-Image.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
以下是我们应用 3D 效果后得到的结果图像。
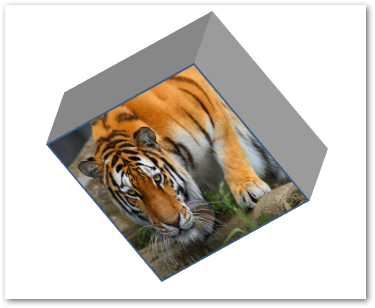
获得免费许可证
您可以获得 免费临时许可证 以使用 Aspose.Slides for Java,而不受评估限制。
结论
在本文中,您学习了如何使用 Java 在 PowerPoint PPT/PPTX 中应用 3D 效果。在代码示例的帮助下,我们演示了如何创建 3D 文本或形状并将 3D 效果应用于 PPT 或 PPTX 演示文稿中的图像。您可以访问 文档 以探索更多关于 Aspose.Slides for Java 的信息。此外,您可以将您的问题或疑问发布到我们的 论坛。