在本文中,您将学习如何使用 Java 动态设置 PowerPoint 演示文稿中的幻灯片背景。更准确地说,本文将介绍如何设置普通幻灯片和母版幻灯片的背景。
用于在 PowerPoint 中设置幻灯片背景的 Java API
要在 PPTX/PPT 演示文稿中设置幻灯片的背景,我们将使用 Aspose.Slides for Java。该 API 允许您从 Java 应用程序中创建、操作和转换 PowerPoint 和 OpenOffice 演示文稿。您可以 下载 API 或使用以下配置将其安装在基于 Maven 的应用程序中。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-slides</artifactId>
<version>21.8</version>
<classifier>jdk16</classifier>
</dependency>
在 Java 中设置普通幻灯片的背景颜色
以下是使用 Java 在 PowerPoint 演示文稿中设置普通幻灯片的背景颜色的步骤。
- 首先,使用 Presentation 类加载 PowerPoint 演示文稿。
- 然后,通过指定其索引设置所需幻灯片的背景,例如背景类型、颜色、填充类型等。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存更新的演示文稿。
下面的代码示例演示如何在 PowerPoint 演示文稿中设置幻灯片的背景。
// 实例化表示演示文件的 Presentation 类
Presentation pres = new Presentation("presentation.pptx");
try {
// 将第一个 ISlide 的背景颜色设置为蓝色
pres.getSlides().get_Item(0).getBackground().setType(BackgroundType.OwnBackground);
pres.getSlides().get_Item(0).getBackground().getFillFormat().setFillType(FillType.Solid);
pres.getSlides().get_Item(0).getBackground().getFillFormat().getSolidFillColor().setColor(Color.BLUE);
// 保存演示文稿
pres.save("ContentBG.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
设置背景前的幻灯片截图如下。
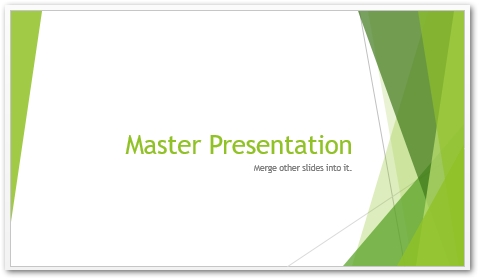
下面是设置背景后的 PowerPoint 幻灯片。

在 Java 中设置母版幻灯片的背景颜色
您还可以设置将影响演示文稿中所有幻灯片的母版幻灯片的背景。以下是更改母版幻灯片背景颜色的步骤。
- 首先,使用 Presentation 类加载 PowerPoint 演示文稿。
- 然后,设置母版幻灯片的背景,例如背景类型、颜色、填充类型等。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存更新的演示文稿。
下面的代码示例演示如何在 PowerPoint 中更改母版幻灯片的背景。
// 实例化表示演示文件的 Presentation 类
Presentation pres = new Presentation("presentation.pptx");
try {
// 将 Master ISlide 的背景颜色设置为绿色
pres.getMasters().get_Item(0).getBackground().setType(BackgroundType.OwnBackground);
pres.getMasters().get_Item(0).getBackground().getFillFormat().setFillType(FillType.Solid);
pres.getMasters().get_Item(0).getBackground().getFillFormat().getSolidFillColor().setColor(Color.GREEN);
// 保存演示文稿
pres.save("MasterBG.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
设置幻灯片的渐变背景颜色
以下是在 PowerPoint 演示文稿中设置幻灯片渐变背景颜色的步骤。
- 首先,使用 Presentation 类加载 PowerPoint 演示文稿。
- 将 Presentation.getSlides().getItem(0).getBackground().setType() 设置为 FillType.Gradient。
- 将 TileFlip 设置为 TileFlip.FlipBoth。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存更新的演示文稿。
下面的代码示例展示了如何在 PowerPoint 中设置幻灯片的渐变背景颜色。
// 实例化表示演示文件的 Presentation 类
Presentation pres = new Presentation("presentation.pptx");
try {
// 将渐变效果应用于背景
pres.getSlides().get_Item(0).getBackground().setType(BackgroundType.OwnBackground);
pres.getSlides().get_Item(0).getBackground().getFillFormat().setFillType(FillType.Gradient);
pres.getSlides().get_Item(0).getBackground().getFillFormat().getGradientFormat().setTileFlip(TileFlip.FlipBoth);
// 保存演示文稿
pres.save("ContentBG_Grad.pptx", SaveFormat.Pptx);
} finally {
if (pres != null) pres.dispose();
}
以下屏幕截图显示了幻灯片的渐变背景。
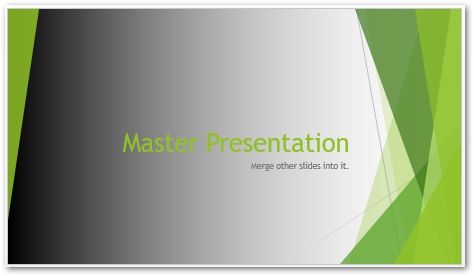
使用 Java 将图像设置为幻灯片背景
以下是使用 Java 将图像设置为幻灯片背景的步骤。
- 首先,使用 Presentation 类加载 PowerPoint 演示文稿。
- 将 FillType 设置为 FillType.Picture。
- 将 PictureFillMode 设置为 PictureFillMode.Stretch。
- 将图像添加到演示文稿的集合中,并将其引用到 IPPImage 对象中。
- 使用 setImage(IPPImage) 方法将图像设置为背景。
- 最后,使用 Presentation.save(String, SaveFormat) 方法保存更新的演示文稿。
以下代码示例演示如何将图像设置为 PowerPoint 演示文稿中幻灯片的背景。
// 实例化表示演示文件的 Presentation 类
Presentation pres = new Presentation();
try {
// 用图像设置背景
pres.getSlides().get_Item(0).getBackground().setType(BackgroundType.OwnBackground);
pres.getSlides().get_Item(0).getBackground().getFillFormat().setFillType(FillType.Picture);
pres.getSlides().get_Item(0).getBackground().getFillFormat().getPictureFillFormat()
.setPictureFillMode(PictureFillMode.Stretch);
// 设置图片
IPPImage imgx = pres.getImages().addImage(Files.readAllBytes(Paths.get("Desert.jpg")));
// 将图像添加到演示文稿的图像集合
pres.getSlides().get_Item(0).getBackground().getFillFormat().getPictureFillFormat().getPicture().setImage(imgx);
// 保存演示文稿
pres.save("ContentBG_Img.pptx", SaveFormat.Pptx);
} catch (IOException e) {
} finally {
if (pres != null) pres.dispose();
}
获取免费 API 许可证
您可以通过请求 临时许可 来使用 Aspose.Slides for Java,而不受评估限制。
结论
在本文中,您学习了如何使用 Java 在 PowerPoint PPTX 或 PPT 中设置幻灯片的背景。此外,您还了解了如何设置 PowerPoint 演示文稿的渐变或图像背景。您可以访问 文档 来探索 Aspose.Slides for Java 的其他功能。此外,您可以随时通过我们的 论坛 让我们知道您的查询。