
MS Project 是一款流行的项目管理软件,广泛用于有效地组织、管理和跟踪项目活动。它允许创建任务、添加资源、将任务分配给资源、监控进度以及管理与预算相关的活动。甘特图视图是项目的默认视图。它列出了项目任务并显示了它们之间的关系。它还使用甘特条显示项目的时间表。在本文中,我们将学习如何使用 Java 阅读 MS 项目的甘特图。
本文将涵盖以下主题:
- Microsoft Project 中的甘特图是什么
- Java API 读取项目甘特图
- 阅读甘特图视图并检索条形样式
- 阅读甘特图视图的网格线
- 提取甘特图视图的文本样式
- 检索甘特图视图的进度线
- 阅读底部时间刻度层
- 读取中间时间刻度层
- 检索顶级时间刻度层
Microsoft Project 中的甘特图是什么
甘特图是一种显示项目进度的条形图。它是项目任务与时间的关系的图形表示,并提供了整个项目的鸟瞰图。 Microsoft Project 中的甘特图视图显示以下内容:
- 项目进度
- 时间估计
- 项目资源和团队成员
- 任务优先级
- 任务依赖
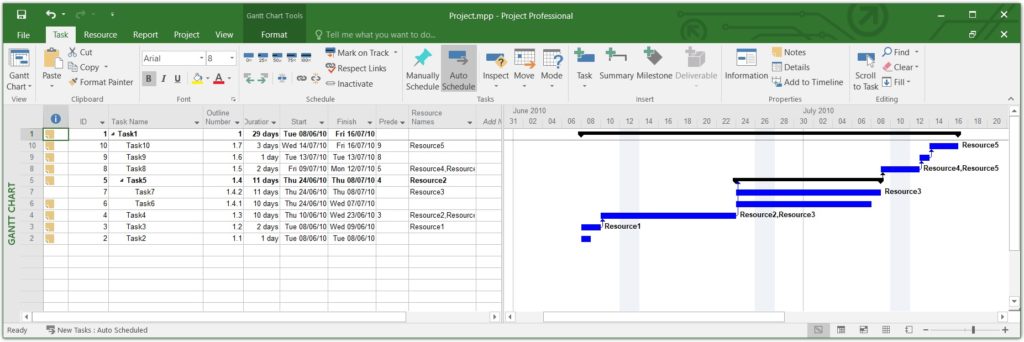
Java API 读取项目甘特图
为了从 MPP 文件中读取项目的甘特图视图,我们将使用 Aspose.Tasks for Java API。它允许在 Java 应用程序中以编程方式创建、编辑或操作 Microsoft Project 文件。 API 的 Project 类代表一个项目。它是公开各种方法以执行不同功能的主要类。它还允许读取受支持的项目管理格式之一,例如 MPP、MPT、MPX 和 XML。 API 的 GanttChartView 类表示甘特图视图。它公开了以编程方式处理甘特图的各种属性和方法。
请下载 API 的 JAR 或在基于 Maven 的 Java 应用程序中添加以下 pom.xml 配置。
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>http://repository.aspose.com/repo/</url>
</repository>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-tasks</artifactId>
<version>22.5</version>
<classifier>jdk18</classifier>
</dependency>
阅读甘特图视图并检索条形样式
API 的 GanttBarStyle 类表示 MS Project 在甘特图视图中使用的条形样式。我们可以按照以下步骤阅读甘特图视图并检索条形样式:
- 首先,使用 Project 类加载项目文件。
- 接下来,通过索引从 ViewCollection 中获取默认视图。
- 然后,创建 GanttChartView 类和类型转换视图的实例。
- (可选)读取基本属性并显示数据。
- 之后,使用 getBarStyles() 获取甘特图视图的条形样式列表。
- 最后,将条形样式作为 GanttBarStyle 类对象循环并显示值。
以下代码示例显示了如何在 Java 中读取甘特图视图的条形样式。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
View view = project.getViews().toList().get(0);
GanttChartView gcView = (GanttChartView) view;
// 显示基本信息
System.out.println("Bar Rounding: " + gcView.getBarRounding());
System.out.println("Show Bar Splits: " + gcView.getShowBarSplits());
System.out.println("Show Drawings: " + gcView.getShowDrawings());
System.out.println("RollUp Gantt Bars: " + gcView.getRollUpGanttBars());
System.out.println("Hide Rollup Bars When Summary Expanded: " + gcView.getHideRollupBarsWhenSummaryExpanded());
System.out.println("Bar Size: " + gcView.getBarSize());
System.out.println("Bar Styles count: " + gcView.getBarStyles().size());
System.out.println("-----------------------------------------------");
// 显示栏样式
for (GanttBarStyle barStyle : gcView.getBarStyles())
{
System.out.println("Row: " + barStyle.getRow());
System.out.println("Name: " + barStyle.getName());
System.out.println("ShowFor: " + barStyle.getShowForTaskUid());
System.out.println("From:" + getbarStylesFromToName(barStyle.getFrom()));
System.out.println("To:" + getbarStylesFromToName(barStyle.getTo()));
System.out.println("Middle Shape:" + getbarStylesMiddleShapeName(barStyle.getMiddleShape()));
System.out.println("Middle Shape Color:" + barStyle.getMiddleShapeColor());
System.out.println("Start Shape:" + getbarStylesShapeName(barStyle.getStartShape()));
System.out.println("End Shape:" + getbarStylesShapeName(barStyle.getEndShape()));
System.out.println("End Shape Color:" + barStyle.getEndShapeColor());
System.out.println("-----------------------------------------------");
}
}
// 此函数返回字段名称
private static String getbarStylesFromToName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = Field.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
// 此函数返回中间形状名称
private static String getbarStylesMiddleShapeName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = GanttBarMiddleShape.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
// 此函数返回开始或结束形状名称
private static String getbarStylesShapeName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = GanttBarEndShape.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
Bar Rounding: true
Show Bar Splits: true
Show Drawings: true
RollUp Gantt Bars: false
Hide Rollup Bars When Summary Expanded: false
Bar Size: 3
Bar Styles count: 40
-----------------------------------------------
Row: 1
Name: Task
ShowFor: null
From:TaskStart
To:TaskFinish
Middle Shape:RectangleBar
Middle Shape Color:java.awt.Color[r=0,g=0,b=255]
Start Shape:NoBarEndShape
End Shape:NoBarEndShape
End Shape Color:java.awt.Color[r=0,g=0,b=0]
-----------------------------------------------
...
在 Java 中读取甘特图视图的网格线
API 的 Gridlines 类表示出现在甘特图视图中的网格线。我们可以按照以下步骤读取网格线的颜色、间隔、图案和类型:
- 首先,使用 Project 类加载项目文件。
- 接下来,通过索引从 ViewCollection 中获取默认视图。
- 然后,创建 GanttChartView 类和类型转换视图的实例。
- 之后,使用 getGridlines() 获取甘特图视图的网格线列表。
- 最后,将网格线作为 Gridlines 类对象循环并显示值。
以下代码示例展示了如何在 Java 中读取甘特图视图的网格线。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示网格线信息
System.out.println("Gridlines Count: " + gcView.getGridlines().size());
Gridlines gridlines = gcView.getGridlines().get(0);
System.out.println("Gridlines Type: " + gridlines.getType());
System.out.println("Gridlines Interval: " + gridlines.getInterval());
System.out.println("Gridlines NormalColor: " + gridlines.getNormalColor());
System.out.println("Gridlines NormalPattern: " + getLinePatternName(gridlines.getNormalPattern()));
System.out.println("Gridlines IntervalPattern: " +gridlines.getIntervalPattern());
System.out.println("Gridlines IntervalColor: " + gridlines.getIntervalColor());
}
// 此函数返回线模式名称
private static String getLinePatternName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = LinePattern.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
Gridlines Count: 14
Gridlines Type: 3
Gridlines Interval: 0
Gridlines NormalColor: java.awt.Color[r=192,g=192,b=192]
Gridlines NormalPattern: Solid
Gridlines IntervalPattern: 0
Gridlines IntervalColor: java.awt.Color[r=192,g=192,b=192]
在Java中提取甘特图视图的文本样式
API 的 TextStyle 类表示甘特图视图中项目的文本的视觉样式。我们可以按照以下步骤读取颜色、背景颜色、字体和字体样式:
- 首先,使用 Project 类加载项目文件。
- 接下来,通过索引从 ViewCollection 中获取默认视图。
- 然后,创建 GanttChartView 类和类型转换视图的实例。
- 之后,使用 getTextStyles() 获取甘特图视图的文本样式列表。
- 最后,为第一个文本样式初始化 TextStyle 类对象并显示值。
以下代码示例显示了如何在 Java 中读取甘特图视图的文本样式。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示文本样式信息
System.out.println("Text Styles Count: " + gcView.getTextStyles().size());
TextStyle textStyle = gcView.getTextStyles().get(0);
System.out.println("Background Color: " + textStyle.getBackgroundColor());
System.out.println("Text Color: " + textStyle.getColor());
System.out.println("Font Family: " + textStyle.getFont().getFontFamily());
System.out.println("Font Size: " + textStyle.getFont().getSize());
System.out.println("Font Style: " + getFontStyleName(textStyle.getFont().getStyle()));
}
// 此函数返回字体样式名称
private static String getFontStyleName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = FontStyles.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
Text Styles Count: 19
Background Color: java.awt.Color[r=0,g=0,b=0]
Text Color: java.awt.Color[r=0,g=0,b=255]
Font Family: Arial
Font Size: 8.0
Font Style: Regular
在 Java 中检索甘特图视图的进度线
进度线出现在甘特图视图中,显示任务是否落后或按时完成。我们可以按照下面给出的步骤读取进度线的各种属性:
- 首先,使用 Project 类加载项目文件。
- 接下来,通过索引从 ViewCollection 中获取默认视图。
- 然后,创建 GanttChartView 类和类型转换视图的实例。
- 之后,使用 getProgressLines() 获取甘特图视图的进度线列表。
- 最后,显示进度线属性值。
以下代码示例显示了如何在 Java 中读取甘特图视图的进度线。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示进度线信息
System.out.println("ProgressLines.BeginAtDate: " + gcView.getProgressLines().getBeginAtDate().toString());
System.out.println("ProgressLines.isBaselinePlan: " + gcView.getProgressLines().isBaselinePlan());
System.out.println( "ProgressLines.DisplaySelected: " + gcView.getProgressLines().getDisplaySelected());
System.out.println("ProgressLines.SelectedDates.Count: " + gcView.getProgressLines().getSelectedDates().size());
System.out.println("ProgressLines.DisplayAtRecurringIntervals: " + gcView.getProgressLines().getDisplayAtRecurringIntervals());
System.out.println("ProgressLines.RecurringInterval.Interval: " + gcView.getProgressLines().getRecurringInterval().getInterval() );
System.out.println("ProgressLines.RecurringInterval.WeeklyDays.Count" + gcView.getProgressLines().getRecurringInterval().getWeeklyDays().size());
System.out.println("RecurringInterval.DayType.Friday: " + (int) gcView.getProgressLines().getRecurringInterval().getWeeklyDays().get(1));
System.out.println("RecurringInterval.DayType.Saturday: " + (int)gcView.getProgressLines().getRecurringInterval().getWeeklyDays().get(2));
System.out.println("RecurringInterval.DayType.Sunday: " + (int)gcView.getProgressLines().getRecurringInterval().getWeeklyDays().get(0));
System.out.println("ProgressLines.ShowDate" + gcView.getProgressLines().getShowDate());
System.out.println("ProgressLines.ProgressPointShape: " + gcView.getProgressLines().getProgressPointShape());
System.out.println("ProgressLines.ProgressPointColor: " + gcView.getProgressLines().getProgressPointColor());
System.out.println("ProgressLines.LineColor: " + gcView.getProgressLines().getLineColor());
System.out.println("ProgressLines.LinePattern" + gcView.getProgressLines().getLinePattern());
System.out.println("ProgressLines.OtherProgressPointShape: " + gcView.getProgressLines().getOtherProgressPointShape());
System.out.println("ProgressLines.OtherProgressPointColor: " + gcView.getProgressLines().getOtherProgressPointColor().toString());
System.out.println("ProgressLines.OtherLineColor: " + gcView.getProgressLines().getOtherLineColor());
}
阅读 Java 中的底层时间刻度层
时间刻度显示时间单位、天、月、日历年或会计年度。项目视图中有三个 timescale tiers 来显示每个层的时间尺度值,例如计数、单位、标签、对齐方式等。我们可以按照以下给出的步骤读取底部的时间尺度层:
- 首先,使用 Project 类加载项目文件。
- 接下来,通过索引从 ViewCollection 中获取默认视图。
- 然后,创建 GanttChartView 类和类型转换视图的实例。
- 之后,使用 getBottomTimescaleTier() 获取视图底部时间刻度层的设置。
- 最后,显示底部时间刻度层值。
以下代码示例显示了如何在 Java 中读取甘特图视图的底部时间刻度层。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示底部时间刻度层信息
System.out.println("BottomTimescaleTier Count: " + gcView.getBottomTimescaleTier().getCount());
System.out.println("BottomTimescaleTier Unit: " + getTimescaleUnitName(gcView.getBottomTimescaleTier().getUnit()));
System.out.println("BottomTimescaleTier UsesFiscalYear: " + gcView.getBottomTimescaleTier().getUsesFiscalYear());
System.out.println("BottomTimescaleTier Alignment: " + getAlignmentName(gcView.getBottomTimescaleTier().getAlignment()));
System.out.println("BottomTimescaleTier ShowTicks: " + gcView.getBottomTimescaleTier().getShowTicks());
System.out.println("BottomTimescaleTier Label: " + getDateLabelName(gcView.getBottomTimescaleTier().getLabel()));
}
// 此函数返回时间刻度数据标签名称
private static String getDateLabelName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = DateLabel.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
// 此函数返回对齐标题
private static String getAlignmentName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = StringAlignment.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
// 此函数返回时间刻度单位名称
private static String getTimescaleUnitName(int val) throws IllegalArgumentException, IllegalAccessException {
String name = null;
java.lang.reflect.Field[] fields = TimescaleUnit.class.getDeclaredFields();
for (java.lang.reflect.Field f : fields) {
int fVal = f.getInt(f);
if( fVal == val)
{
name = f.getName();
}
}
return name;
}
BottomTimescaleTier Count: 2
BottomTimescaleTier Unit: Days
BottomTimescaleTier UsesFiscalYear: true
BottomTimescaleTier Alignment: Center
BottomTimescaleTier ShowTicks: true
BottomTimescaleTier Label: DayOfMonthDd
阅读 Java 中的中间时间尺度层
同样,我们可以按照前面提到的步骤读取中间时间尺度层。但是,我们将在步骤 4 中使用 getMiddleTimescaleTier() 获取视图的中间时间刻度层的设置。
以下代码示例显示了如何在 Java 中读取甘特图视图的中间时间刻度层。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示中间时间尺度层信息
System.out.println("MiddleTimescaleTier Count: " + gcView.getMiddleTimescaleTier().getCount());
System.out.println("TimescaleUnit Weeks: " + getTimescaleUnitName(gcView.getMiddleTimescaleTier().getUnit()));
System.out.println("MiddleTimescaleTier Alignment: " + getAlignmentName(gcView.getMiddleTimescaleTier().getAlignment()));
System.out.println("MiddleTimescaleTier ShowTicks: " + gcView.getMiddleTimescaleTier().getShowTicks());
System.out.println("MiddleTimescaleTier Label: " + getDateLabelName(gcView.getMiddleTimescaleTier().getLabel()));
}
MiddleTimescaleTier.Count: 1
TimescaleUnit.Weeks: Months
MiddleTimescaleTier.Alignment: Center
MiddleTimescaleTier.ShowTicks: true
MiddleTimescaleTier.Label: MonthMmmmYyyy
检索 Java 中的顶级时间刻度层
我们还可以按照前面提到的步骤读取顶级时间尺度层。但是,我们将在第 4 步中使用 getTopTimescaleTier() 获取视图顶部时间刻度层的设置。
以下代码示例显示了如何在 Java 中读取甘特图视图的顶部时间刻度层。
public static void main(String[] args) throws Exception
{
// 文档目录的路径。
String dataDir = "C:\\Files\\Tasks\\";
// 加载项目
Project project = new Project(dataDir + "Project.mpp");
// 获取默认视图为 GanttChartView
GanttChartView gcView = (GanttChartView) project.getViews().toList().get(0);
// 显示顶级时间刻度层信息
System.out.println("TopTimescaleTier Unit: " + getTimescaleUnitName(gcView.getTopTimescaleTier().getUnit()));
System.out.println("TopTimescaleTier UsesFiscalYear: " + gcView.getTopTimescaleTier().getUsesFiscalYear() );
System.out.println("TopTimescaleTier Alignment: " + getAlignmentName(gcView.getTopTimescaleTier().getAlignment()));
System.out.println("TopTimescaleTier ShowTicks: " + gcView.getTopTimescaleTier().getShowTicks());
System.out.println("TopTimescaleTier Label" + getDateLabelName(gcView.getTopTimescaleTier().getLabel()));
}
TopTimescaleTier Unit: Quarters
TopTimescaleTier UsesFiscalYear: true
TopTimescaleTier Alignment: Center
TopTimescaleTier ShowTicks: true
TopTimescaleTier Label: QuarterQtrQYyyy
获得免费许可证
您可以 获得免费的临时许可证 试用该库而不受评估限制。
结论
在本文中,我们学习了如何
- 在 Java 中以编程方式阅读甘特图视图;
- 提取甘特图视图的条形样式和文本样式;
- 检索甘特图视图的网格线和进度线;
- 使用 Java 读取甘特图视图的时间刻度单位。
此外,您可以使用 documentation 了解有关 Aspose.Tasks for Java API 的更多信息。如有任何歧义,请随时在 论坛 上与我们联系。